The Solid Principles
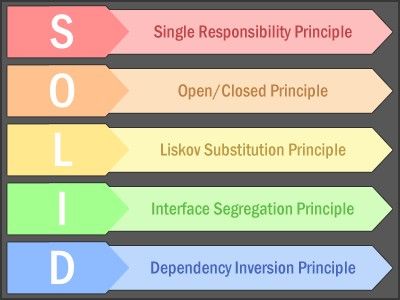
Cotinuing the saga in OOP Principles today I'll talk about SOLID.
SOLID is some principles to writing better codes, it increases code cohesion and decreases coupling. But first what means cohesion and what means coupling.
- Cohesion is basically sticking together. Imagine a watch, all the gears have cohesion, they move together, each gear moves independently and at the same time together. That's cohesion.
- Coupling is the interdependence between the class and modules. In software develepment the less coupled the classes are, better. Because is easy to maintain, test, and modify without affecting other parts of the software. There is a joke that I love for very coupled codes, We said it's a Spaghetti Code, everything is so together that is almost impossible to isolate it.
Now that you know what cohesion and coupling means let's see what is SOLID.
Single Responsability Principle
This principle says that a class should have one and only one reason to exist and change. This is the easiest one. A class should not get the form imput, validate, check permission, manipulate the database, and render the view. In this example we should have many classes, each one doing only one thing. Avoid "Swiss Army Knife Classes".
Open-Closed Principle
The open-closed principle says: "A class should be open for extension and closed for modifications". It tells you to write your code so that you will be able to add new functionality without changing the existing code. Let me give you a better example. Imagine everytime you want to plug a new USB device you need to recode the BIOS. Nowadays most of the external devices are plug-and-play, and your OS recognize it without needing the driver. I remember a day, long time ago, after formating a Win98. It didn't reconize the lan board because it was needing the driver, so I put the drivers on a flash drive and plugged it. It also wasn't recognized. I needed to record a CD with the drivers to make everything works.
Liskhov Substitution Principle
The principle defines that instances of a superclass should be replaceable with instances of its subclasses without breaking the application.
Interface Segregation Principle
The Interface Segregation Principles says that is better to have many small interfaces than a few large ones. This is directly related to coupling. With the example above is easy to understand why is better to use small interfaces.
Dependecy Inversion Principle
Dependency Inversion says that is better to depend on abstraction than implemented classes. This is useful because if your dependencies are on abstract classes the risk of the changes lower. You depend of the behavior's idea not the behavior itself and in case of changes, you don't need to refactor all the code, you just change the implementation.
I hope you've enjoyed this post, and that I helped clarifying the Solid Principles to you.
17/02/2020